Bash If-Then-Else Example
This is a Bash If-Then-Else Example. Sometimes you need to specify different courses of action to be taken in a shell script, depending on the success or failure of a command. The if construction allows you to specify such conditions.
The following table shows an overview of the whole article:
Table Of Contents
1. Introduction
The most compact syntax of the if command is:
if TEST-COMMANDS; then CONSEQUENT-COMMANDS; else ALTERNATE-CONSEQUENT-COMMANDS; fi
The TEST-COMMAND
list is executed, and if its return status is zero, the CONSEQUENT-COMMANDS
list is executed. The return status is the exit status of the last command executed, or zero if no condition tested true.
The TEST-COMMAND
often involves numerical or string comparison tests, but it can also be any command that returns a status of zero when it succeeds and some other status when it fails. Unary expressions are often used to examine the status of a file. If the FILE argument to one of the primaries is of the form /dev/fd/N
, then file descriptor “N” is checked. stdin, stdout and stderr and their respective file descriptors may also be used for tests.
The table below contains an overview of the so-called “primaries” that make up the TEST-COMMAND
command or list of commands. These primaries are put between square brackets to indicate the test of a conditional expression.
Primary | Meaning |
---|---|
[ -a FILE ] | True if FILE exists. |
[ -b FILE ] | True if FILE exists and is a block-special file. |
[ -c FILE ] | True if FILE exists and is a character-special file. |
[ -d FILE ] | True if FILE exists and is a directory. |
[ -e FILE ] | True if FILE exists. |
[ -f FILE ] | True if FILE exists and is a regular file. |
[ -g FILE ] | True if FILE exists and its SGID bit is set. |
[ -h FILE ] | True if FILE exists and is a symbolic link. |
[ -k FILE ] | True if FILE exists and its sticky bit is set. |
[ -p FILE ] | True if FILE exists and is a named pipe (FIFO). |
[ -r FILE ] | True if FILE exists and is readable. |
[ -s FILE ] | True if FILE exists and has a size greater than zero. |
[ -t FD ] | True if file descriptor FD is open and refers to a terminal. |
[ -u FILE ] | True if FILE exists and its SUID (set user ID) bit is set. |
[ -w FILE ] | True if FILE exists and is writable. |
[ -x FILE ] | True if FILE exists and is executable. |
[ -O FILE ] | True if FILE exists and is owned by the effective user ID. |
[ -G FILE ] | True if FILE exists and is owned by the effective group ID. |
[ -L FILE ] | True if FILE exists and is a symbolic link. |
[ -N FILE ] | True if FILE exists and has been modified since it was last read. |
[ -S FILE ] | True if FILE exists and is a socket. |
[ FILE1 -nt FILE2 ] | True if FILE1 has been changed more recently than FILE2, or if FILE1 exists and FILE2 does not. |
[ FILE1 -ot FILE2 ] | True if FILE1 is older than FILE2, or is FILE2 exists and FILE1 does not. |
[ FILE1 -ef FILE2 ] | True if FILE1 and FILE2 refer to the same device and inode numbers. |
[ -o OPTIONNAME ] | True if shell option “OPTIONNAME” is enabled. |
[ -z STRING ] | True of the length if “STRING” is zero. |
[ -n STRING ] or [ STRING ] | True if the length of “STRING” is non-zero. |
[ STRING1 == STRING2 ] | True if the strings are equal. “=” may be used instead of “==” for strict POSIX compliance. |
[ STRING1 != STRING2 ] | True if the strings are not equal. |
[ STRING1 < STRING2 ] | True if “STRING1” sorts before “STRING2” lexicographically in the current locale. |
[ STRING1 > STRING2 ] | True if “STRING1” sorts after “STRING2” lexicographically in the current locale. |
[ ARG1 OP ARG2 ] | “OP” is one of -eq, -ne, -lt, -le, -gt or -ge. These arithmetic binary operators return true if “ARG1” is equal to, not equal to, less than, less than or equal to, greater than, or greater than or equal to “ARG2”, respectively. “ARG1” and “ARG2” are integers. |
Expressions may be combined using the following operators, listed in decreasing order of precedence:
Operation | Effect |
---|---|
[ ! EXPR ] | True if EXPR is false. |
[ ( EXPR ) ] | Returns the value of EXPR. This may be used to override the normal precedence of operators. |
[ EXPR1 -a EXPR2 ] | True if both EXPR1 and EXPR2 are true. |
[ EXPR1 -o EXPR2 ] | True if either EXPR1 or EXPR2 is true. |
The [ (or test) built-in evaluates conditional expressions using a set of rules based on the number of arguments. More information about this subject can be found in the Bash documentation.
Just like the if is closed with fi, the opening square bracket should be closed after the conditions have been listed.
The CONSEQUENT-COMMANDS
list that follows the then statement can be any valid UNIX command, any executable program, any executable shell script or any shell statement, with the exception of the closing fi.
The ALTERNATE-CONSEQUENT-COMMANDS
list following the else statement can hold any UNIX-style command that returns an exit status.
It is important to remember that the then and fi are considered to be separated statements in the shell.
Therefore, when issued on the command line, they are separated by a semi-colon. In a script, the different parts of the if statement are usually well-separated.
2. Expressions used with if-then-else
1.1 Checking Files
The following file represents a simple textfile for some tests:
Test1.txt
1:Andreas 2:Marcus 3:Tom 4:Steve
This is the construct to use to take one course of action if the if commands test true, and another if it tests false.
ifelse1.sh
echo "Checking..." grep Hardy Test1.txt if [ $? -ne 0 ] then echo 'Hardy not found in File Test1.txt!' else echo 'Hardy found in File Test1.txt!' fi echo echo "...done."
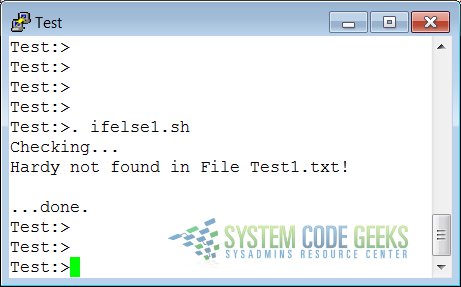
ifelse2.sh
echo "Checking..." grep Tom Test1.txt if [ $? -ne 0 ] then echo 'Tom not found in File Test1.txt!' else echo 'Tom found in File Test1.txt!' fi echo echo "...done."
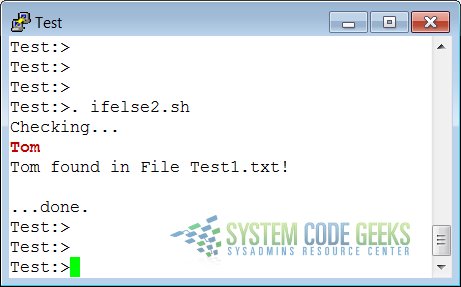
1.2 Checking command line arguments
Instead of setting a variable and then executing a script, it is frequently more elegant to put the values for the variables on the command line.
We use the positional parameters $1, $2, ..., $N
for this purpose. $#
refers to the number of command line arguments. $0
refers to the name of the script.
The following is a simple example:
ifelse3.sh
echo "Checking..." grep $1 Test1.txt if [ $? -ne 0 ] then echo $1 'not found in File Test1.txt!' else echo $1 'found in File Test1.txt!' fi echo echo "...done."
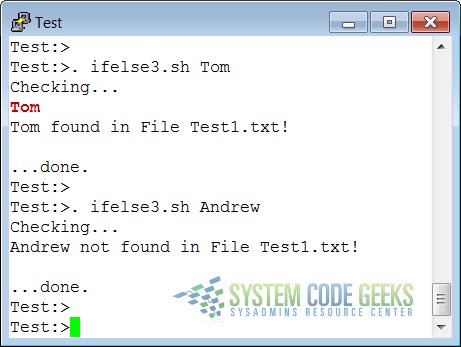
Here’s another example, using two arguments:
compare1.sh
#!/bin/bash value1="$1" value2="$2" echo "Comparing ..." echo if [ $value1 -le $value2 ] then echo "Parameter2:" $value2 "is greater or equal than Parameter1:" $value1 else echo "Parameter1:" $value1 "is greater than Parameter2:" $value2 fi echo echo "...done."
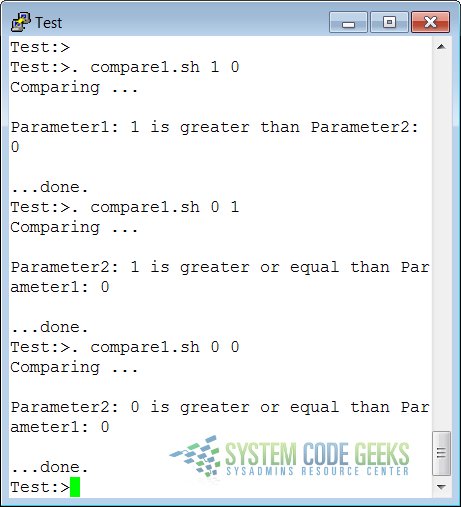
1.3 Testing the number of arguments
The following example shows how to change the previous script so that it prints a message if more or less than 2 arguments are given:
compare2.sh
#!/bin/bash if [ ! $# == 2 ] then echo "Usage: Command Value1 Value2" return 1 fi value1="$1" value2="$2" echo "Comparing ..." echo if [ $value1 -le $value2 ] then echo "Parameter2:" $value2 "is greater or equal than Parameter1:" $value1 else echo "Parameter1:" $value1 "is greater than Parameter2:" $value2 fi echo echo "...done."
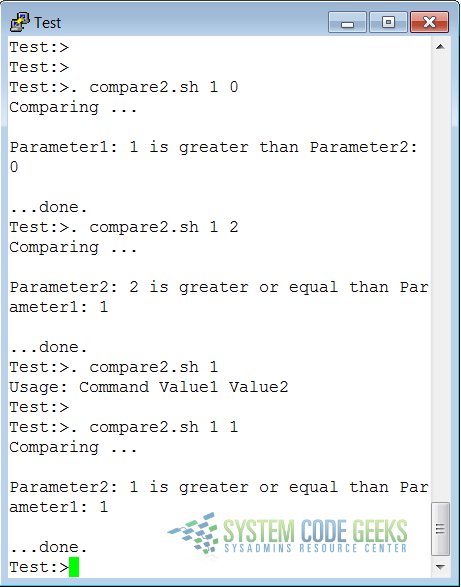
The first argument is referred to as $1
, the second as $2
and so on. The total number of arguments is stored in $#
.
1.4 Testing that a file exists
This test is done in a lot of scripts, because there’s no use in starting a lot of programs if you know they’re not going to work:
fileexists.sh
#!/bin/bash # This script gives information about a file. FILENAME="$1" echo "Properties for $FILENAME:" if [ -f $FILENAME ] then echo "Size is $(ls -lh $FILENAME | awk '{ print $5 }')" echo "Type is $(file $FILENAME | cut -d":" -f2 -)" echo "Inode number is $(ls -i $FILENAME | cut -d" " -f1 -)" else echo "File does not exist." fi
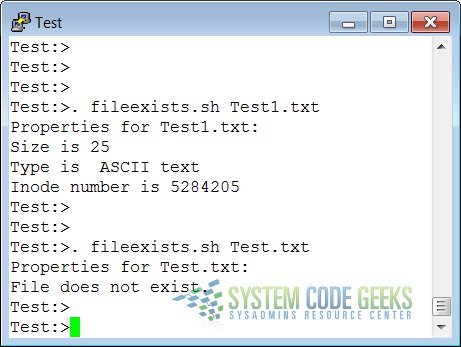
Note that the file is referred to using a variable; in this case it is the first argument to the script. Alternatively, when no arguments are given, file locations are usually stored in variables at the beginning of a script, and their content is referred to using these variables. Thus, when you want to change a file name in a script, you only need to do it once.
2. If-Then-Elif-Else constructs
This is the full form of the if statement:
if TEST-COMMANDS; then CONSEQUENT-COMMANDS; elif MORE-TEST-COMMANDS; then MORE-CONSEQUENT-COMMANDS; else ALTERNATE-CONSEQUENT-COMMANDS; fi
The TEST-COMMANDS
list is executed, and if its return status is zero, the CONSEQUENT-COMMANDS
list is executed.
If TEST-COMMANDS
returns a non-zero status, each elif list is executed in turn, and if its exit status is zero, the corresponding MORE-CONSEQUENT-COMMANDS
is executed and the command completes.
If else is followed by an ALTERNATE-CONSEQUENT-COMMANDS
list, and the final command in the final if or elif clause has a non-zero exit status, then ALTERNATE-CONSEQUENT-COMMANDS
is executed.
The return status is the exit status of the last command executed, or zero if no condition tested true.
Here is a simple example:
compare3.sh
#!/bin/bash value1="$1" value2="$2" echo "Comparing ..." echo if [ $value1 -eq $value2 ] then echo "Parameter2:" $value2 "is equal with Parameter1:" $value1 elif [ $value1 -gt $value2 ] then echo "Parameter1:" $value1 "is greater than Parameter2:" $value2 else echo "Parameter1:" $value1 "is smaller than Parameter2:" $value2 fi echo echo "...done."
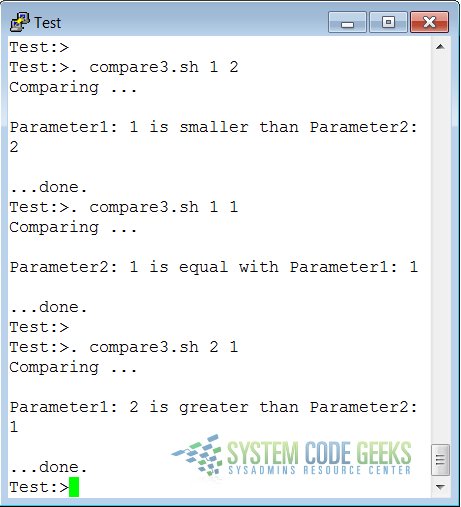
3. Nested If statements
Inside the if statement, you can use another if statement. You may use as many levels of nested ifs as you can logically manage.
This is an example testing leap years:
testleap.sh
#!/bin/bash # This script will test if we're in a leap year or not. year=`date +%Y` if [ $[$year % 400] -eq "0" ] then echo "This is a leap year. February has 29 days." elif [ $[$year % 4] -eq 0 ] then if [ $[$year % 100] -ne 0 ] then echo "This is a leap year, February has 29 days." else echo "This is not a leap year. February has 28 days." fi else echo "This is not a leap year. February has 28 days." fi
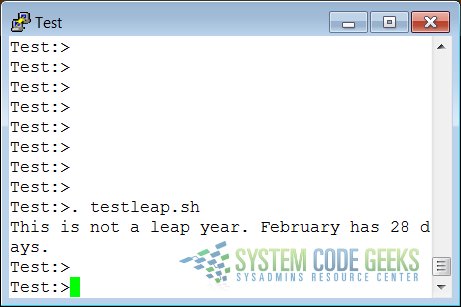
In this example, we use the double brackets for testing an arithmetic expression. This is equivalent to the let statement.
You will get stuck using square brackets here, if you try something like $[$year % 400]
, because here, the square brackets don’t represent an actual command by themselves.
Among other editors, gvim is one of those supporting colour schemes according to the file format. Such editors are useful for detecting errors in your code.
4. Boolean operations
The above compare script can be shortened using the Boolean operators “AND” (&&) and “OR” (||).
compare4.sh
#!/bin/bash value1="$1" value2="$2" echo "Comparing ..." echo if [ $value1 -lt $value2 ] || [ $value1 -eq $value2 ] then echo "Parameter1:" $value2 "is lower or equal than Parameter1:" $value1 else echo "Parameter1:" $value1 "is greater than Parameter2:" $value2 fi echo echo "...done."
5. Using the Exit statement and If
The exit status is most often used if the input requested from the user is incorrect, if a statement did not run successfully or if some other error occurred.
The exit statement takes an optional argument. This argument is the integer exit status code, which is passed back to the parent and stored in the $?
variable.
A zero argument means that the script ran successfully. Any other value may be used by programmers to pass back different messages to the parent, so that different actions can be taken according to failure or success of the child process. If no argument is given to the exit command, the parent shell uses the current value of the $?
variable.
Below is an example with a slightly adapted penguin.sh script, which sends its exit status back to the parent, feed.sh
:
penguin.sh
#!/bin/bash # This script lets you present different menus to Tux. He will only be happy # when given a fish. We've also added a dolphin and (presumably) a camel. if [ "$menu" == "fish" ] then if [ "$animal" == "penguin" ] then echo "Hmmmmmm fish... Tux happy!" elif [ "$animal" == "dolphin" ] then echo "Pweetpeettreetppeterdepweet!" else echo "*prrrrrrrt*" fi else if [ "$animal" == "penguin" ] then echo "Tux don't like that. Tux wants fish!" return 1 elif [ "$animal" == "dolphin" ] then echo "Pweepwishpeeterdepweet!" return 2 else echo "Will you read this sign?!" return 3 fi fi
This script is called upon in the next one, which therefore exports its variables menu and animal:
feed.sh
#!/bin/bash # This script acts upon the exit status given by penguin.sh export menu="$1" export animal="$2" feed="penguin.sh" $feed $menu $animal case $? in 1) echo "Guard: You'd better give'm a fish, less they get violent..." ;; 2) echo "Guard: It's because of people like you that they are leaving earth all the time..." ;; 3) echo "Guard: Buy the food that the Zoo provides for the animals, you ***, how do you think we survive?" ;; *) echo "Guard: Don't forget the guide!" ;; esac
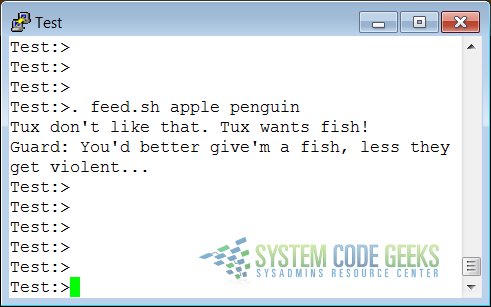
As you can see, exit status codes can be chosen freely. Existing commands usually have a series of defined codes.
6. Using case statements
Nested if statements might be nice, but as soon as you are confronted with a couple of different possible actions to take, they tend to confuse. For the more complex conditionals, use the case syntax:
case EXPRESSION in CASE1) COMMAND-LIST;; CASE2) COMMAND-LIST;; ... CASEN) COMMAND-LIST;; esac
Each case is an expression matching a pattern. The commands in the COMMAND-LIST
for the first match are executed. The “|” symbol is used for separating multiple patterns, and the “)” operator terminates a pattern list.
Each case plus its according commands are called a clause. Each clause must be terminated with “;;”.
Each case statement is ended with the esac statement.
Here is a simple example:
testcase.sh
case "$1" in start) echo "Processes will be started..." ;; stop) echo "Processes will be stopped..." ;; status) echo "Current State of the Processes is..." ;; restart) echo "Processes will be restarted..." ;; *) echo $"Usage: Script {start|stop|restart|status}" esac
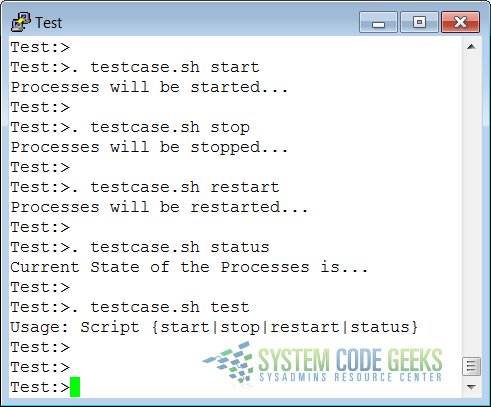
A classical application of such an example is an initscript. This kind of scripts often make use of case statements for starting, stopping and querying system services.
7. Summary
In this chapter we learned how to build complex conditions into our scripts so that different actions can be undertaken upon success or failure of a command. The actions can be determined using the if statement.
More complex definitions of conditions are usually put in a case statement.
Upon successful condition testing, the script can explicitly inform the parent using the exit 0 status.
Upon failure, any other number may be returned. Based on the return code, the parent program can take appropriate action.