Bash If – Statement Example
This is an If – Condition Example. Sometimes you need to specify different courses of action to be taken in a shell script, depending on the success or failure of a command. The if construction allows you to specify such conditions.
The most compact syntax of the if command is:
if TEST-COMMANDS; then CONSEQUENT-COMMANDS; fi
The TEST-COMMAND
list is executed, and if its return status is zero, the CONSEQUENT-COMMANDS
list is executed. The return status is the exit status of the last command executed, or zero if no condition tested true.
The TEST-COMMAND
often involves numerical or string comparison tests, but it can also be any command that returns a status of zero when it succeeds and some other status when it fails. Unary expressions are often used to examine the status of a file. If the FILE argument to one of the primaries is of the form /dev/fd/N
, then file descriptor “N” is checked. stdin, stdout and stderr and their respective file descriptors may also be used for tests.
The following table shows an overview of the whole article:
Table Of Contents
1. Expressions used with if
The table below contains an overview of the so-called “primaries” that make up the TEST-COMMAND
command or list of commands. These primaries are put between square brackets to indicate the test of a conditional expression.
Primary | Meaning |
---|---|
[ -a FILE ] | True if FILE exists. |
[ -b FILE ] | True if FILE exists and is a block-special file. |
[ -c FILE ] | True if FILE exists and is a character-special file. |
[ -d FILE ] | True if FILE exists and is a directory. |
[ -e FILE ] | True if FILE exists. |
[ -f FILE ] | True if FILE exists and is a regular file. |
[ -g FILE ] | True if FILE exists and its SGID bit is set. |
[ -h FILE ] | True if FILE exists and is a symbolic link. |
[ -k FILE ] | True if FILE exists and its sticky bit is set. |
[ -p FILE ] | True if FILE exists and is a named pipe (FIFO). |
[ -r FILE ] | True if FILE exists and is readable. |
[ -s FILE ] | True if FILE exists and has a size greater than zero. |
[ -t FD ] | True if file descriptor FD is open and refers to a terminal. |
[ -u FILE ] | True if FILE exists and its SUID (set user ID) bit is set. |
[ -w FILE ] | True if FILE exists and is writable. |
[ -x FILE ] | True if FILE exists and is executable. |
[ -O FILE ] | True if FILE exists and is owned by the effective user ID. |
[ -G FILE ] | True if FILE exists and is owned by the effective group ID. |
[ -L FILE ] | True if FILE exists and is a symbolic link. |
[ -N FILE ] | True if FILE exists and has been modified since it was last read. |
[ -S FILE ] | True if FILE exists and is a socket. |
[ FILE1 -nt FILE2 ] | True if FILE1 has been changed more recently than FILE2, or if FILE1 exists and FILE2 does not. |
[ FILE1 -ot FILE2 ] | True if FILE1 is older than FILE2, or is FILE2 exists and FILE1 does not. |
[ FILE1 -ef FILE2 ] | True if FILE1 and FILE2 refer to the same device and inode numbers. |
[ -o OPTIONNAME ] | True if shell option “OPTIONNAME” is enabled. |
[ -z STRING ] | True of the length if “STRING” is zero. |
[ -n STRING ] or [ STRING ] | True if the length of “STRING” is non-zero. |
[ STRING1 == STRING2 ] | True if the strings are equal. “=” may be used instead of “==” for strict POSIX compliance. |
[ STRING1 != STRING2 ] | True if the strings are not equal. |
[ STRING1 < STRING2 ] | True if “STRING1” sorts before “STRING2” lexicographically in the current locale. |
[ STRING1 > STRING2 ] | True if “STRING1” sorts after “STRING2” lexicographically in the current locale. |
[ ARG1 OP ARG2 ] | “OP” is one of -eq, -ne, -lt, -le, -gt or -ge. These arithmetic binary operators return true if “ARG1” is equal to, not equal to, less than, less than or equal to, greater than, or greater than or equal to “ARG2”, respectively. “ARG1” and “ARG2” are integers. |
Expressions may be combined using the following operators, listed in decreasing order of precedence:
Operation | Effect |
---|---|
[ ! EXPR ] | True if EXPR is false. |
[ ( EXPR ) ] | Returns the value of EXPR. This may be used to override the normal precedence of operators. |
[ EXPR1 -a EXPR2 ] | True if both EXPR1 and EXPR2 are true. |
[ EXPR1 -o EXPR2 ] | True if either EXPR1 or EXPR2 is true. |
The [ (or test) built-in evaluates conditional expressions using a set of rules based on the number of arguments. More information about this subject can be found in the Bash documentation.
Just like the if is closed with fi, the opening square bracket should be closed after the conditions have been listed.
2. Commands following the then statement
The CONSEQUENT-COMMANDS
list that follows the then statement can be any valid UNIX command, any executable program, any executable shell script or any shell statement, with the exception of the closing fi.
It is important to remember that the then and if are considered to be separated statements in the shell.
Therefore, when issued on the command line, they are separated by a semi-colon. In a script, the different parts of the if statement are usually well-separated.
2.1 Checking Files
The following file represents a simple textfile for some tests:
Test1.txt
1:Andreas 2:Marcus 3:Tom 4:Steve
The first example checks for the existence of a file:
filecheck.sh
#!/bin/bash echo "Checking..." if [ -f Test1.txt ] then echo "File Test1.txt exists." fi echo echo "...done."
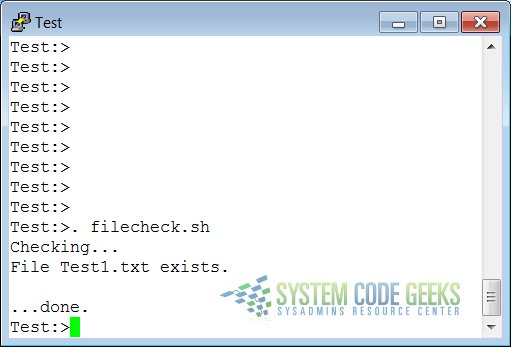
2.2 Testing exit status
The ? variable holds the exit status of the previously executed command (the most recently completed foreground process).
The following example shows a simple test:
exitcheck1.sh
echo "Reading..." ls Test*.txt echo echo "Checking..." if [ $? -eq 0 ] then echo 'Success!' fi echo echo "...done."
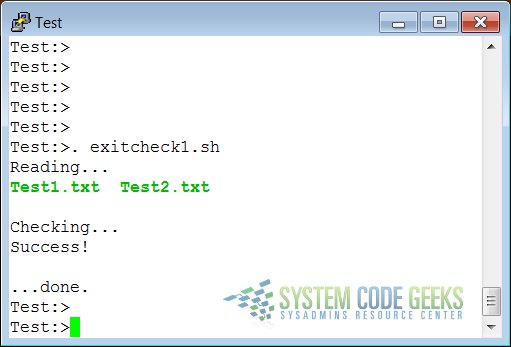
The following example demonstrates that TEST-COMMANDS
might be any UNIX command that returns an exit status, and that if again returns an exit status of zero:
exitcheck2.sh
echo "Checking..." if ! grep Hardy Test1.txt then echo 'Hardy not found in File Test1.txt!' fi echo echo "...done."
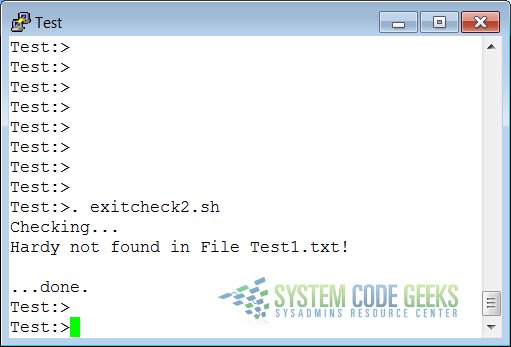
The same result can be obtained as follows:
exitcheck3.sh
echo "Checking..." grep Hardy Test1.txt if [ $? -ne 0 ] then echo 'Hardy not found in File Test1.txt!' fi echo echo "...done."
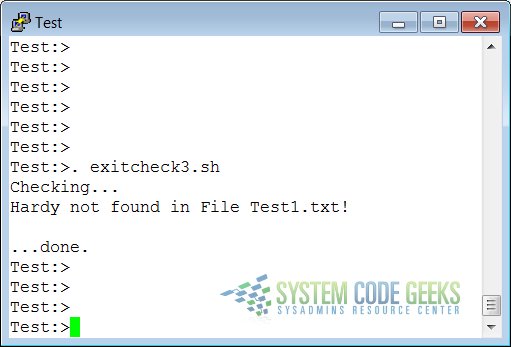
2.3 Numeric comparisons
The examples below use numerical comparisons:
numcomp.sh
echo "Calculating..." num=`ls | wc -l` echo $num echo echo "Checking..." if [ "$num" -lt "100" ] then echo "There exists less then 100 files!" fi echo echo "...done."
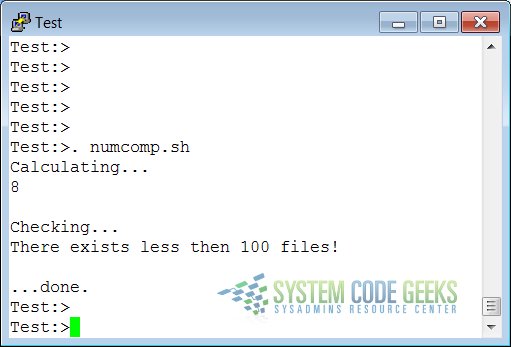
2.4 String comparisons
An example of comparing strings for testing the user ID:
strcomp.sh
echo "Checking..." if [ "$(whoami)" != 'root' ] then echo "You are not logged in as user root." fi echo echo "...done."
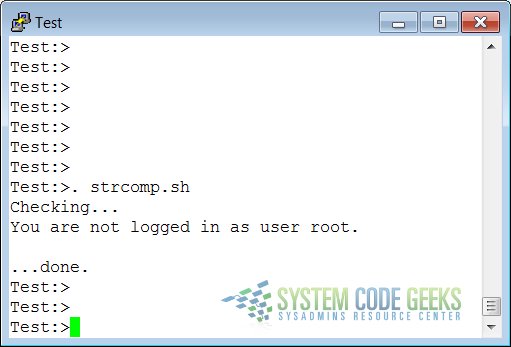
3. Summary
In this chapter we learned how to build conditions into our scripts so that different actions can be undertaken. Upon success or failure of a command. The actions can be determined using the if statement. This allows you to perform arithmetic and string comparisons, and testing of exit code, input and files needed by the script.
The script “exitcheck1.sh” always will show “Success”, because the exit status of the previously executed command “echo Checking…” will run without any problem.